Requirements
The requirements are typical for a TODO list application. User should be able to create multiple TODO lists, remove them if he wants and assign TODOs entries to them. The user can decide whether to move his TODO to trash bin or remove it completely from the system. Each TODO entry should have a title, a description and a due date. The user will also be able to modify a TODO list or a TODO entry. Of course, he can also set TODO entry status to completed or uncompleted. By default newly created TODO entry has uncompleted status. I present these requirements on the following Use case diagram:
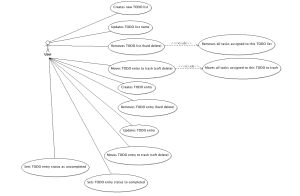
Data model
After defining the requirements, we can design our data model which fits these requirements. Let's take a look at the following database diagram:
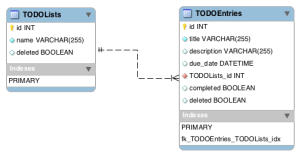
Our data model contains of two tables (entities) TODOLists and TODOEntries with one-to-many relations between them. It means that one TODO list can have many TODO entries. TODOList entity contains tree columns:
- id (not null, autoincrement, primary key, INT field): list identifier
- name (not null, VARCHAR(255) field): name of the list
- deleted (BOOLEAN, FALSE by default field): if TRUE the list is softly deleted (moved to trash bin), otherwise FALSE.
TODOEntries table contains of the following columns:
- id (not null, autoincrement, primary key, INT field): entry identifier
- title (not null, VARCHAR(255) field): task title
- description (null, VARCHAR(255) field): task description
- due_date (null, VARCHAR(255) field): task due date
- TODOLists_id (id, TODOLists foreign key): to which list this entry is assigned
- completed (BOOLEAN, FALSE by default field): if TRUE task was completed, otherwise FALSE
- deleted (BOOLEAN, FALSE by default field): if TRUE entry is softly deleted (moved to trash bin), otherwise FALSE.
Now, when we have the data model, we can create a class diagram of entities in our system.
Class diagram
Entities class diagram looks as follows:
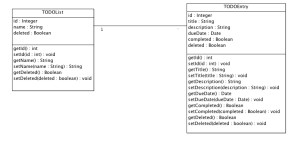
I found that ArgoUML have some problems with exporting diagrams to PNG format. Of course, on the right site of association there is * character. ArgoUML also does not show, which fields and methods are private, public or protected. All attributes in both classes are private and we get and set these values by using setters and getters.
Ok, so now we specified use cases of our system, designed data model and class diagram. In the next article from this series I will go through implementing and testing Hibernate entities. We also need to add additional configuration to our Dynamic Web Project to set additional JPA settings, so that we are able to generate database tables from entities and entities from database tables. Additionally, we need to install MySQL RDBMS and MySQL Java Driver. I will show how to do this in the next article.
Additional information
This article is a part of the series of articles called From Zero to PrimeFaces Hero on JBoss 7.1 with Hibernate, MySQL, Eclipse Indigo and JBoss Tools. If you’d like to get more information about this series, please check out this article. The previous article from this series is “Hello PrimeFaces World !” deployed on JBoss 7.1.
0 comments :: Designing a domain model for a TODO list application
Post a Comment